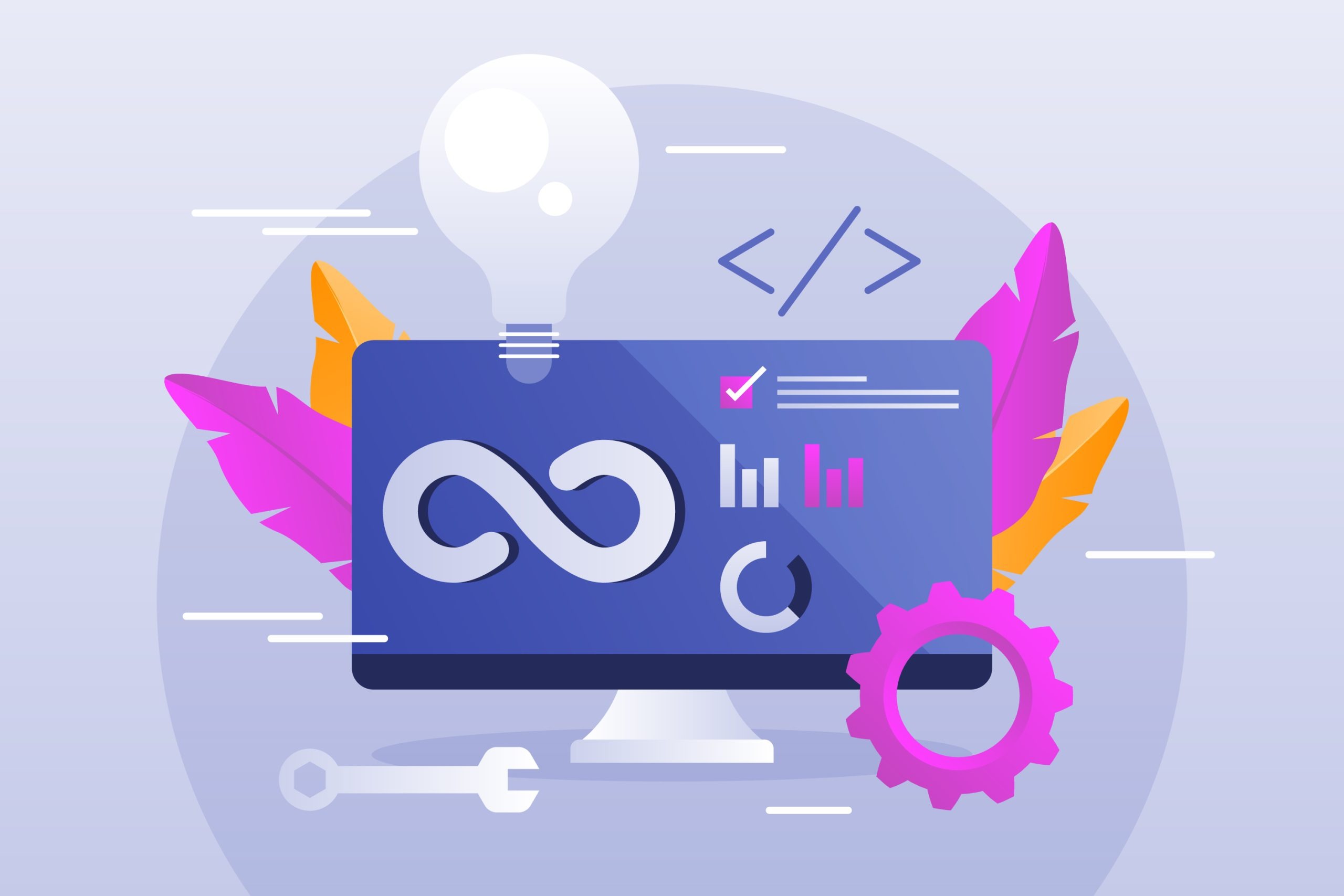
Exploring React Hooks
React Hooks have transformed the way we build React applications by providing a more direct API to the React concepts you already know. Here, we’ll explore the most commonly used hooks, their benefits, and when to use them.
useState
JavaScript
const [state, setState] = useState(initialState);
Explanation: useState allows you to add state to functional components. Use this hook when you need to track simple values like numbers, strings, or objects in your component.
Benefits: Simplifies state management in functional components and eliminates the need for class components for stateful logic.
useEffect
JavaScript
useEffect(() => {
// Side effects here
}, [dependencies]);
Explanation: useEffect is used for side effects in functional components, such as data fetching, subscriptions, or manually changing the DOM. It runs after every render by default, but you can specify when it should run by setting dependencies.
Benefits: Replaces lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount with a unified API.
useContext
JavaScript
const value = useContext(MyContext);
Explanation: useContext lets you subscribe to React context without introducing nesting. Use it when you want to access context data in your component.
Benefits: Makes it easier to share values like themes or user data across the component tree.
useReducer
JavaScript
const [state, dispatch] = useReducer(reducer, initialArg, init);
Explanation: useReducer is usually preferable to useState when you have complex state logic that involves multiple sub-values or when the next state depends on the previous one.
Benefits: Offers a more predictable state transition with the use of actions and reducers, similar to Redux.
useCallback
JavaScript
const memoizedCallback = useCallback(() => {
// Your callback here
}, [dependencies]);
Explanation: useCallback returns a memoized version of the callback that only changes if one of the dependencies has changed. It’s useful when passing callbacks to optimized child components that rely on reference equality to prevent unnecessary renders.
Benefits: Helps in optimizing performance for components that rely on reference equality.
useMemo
JavaScript
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
Explanation: useMemo will only recompute the memoized value when one of the dependencies has changed. This optimization helps to avoid expensive calculations on every render.
Benefits: Reduces computational cost by memoizing values.
useRef
JavaScript
const refContainer = useRef(initialValue);
Explanation: useRef persists values between renders. It can be used to store a mutable value that does not cause a re-render when updated.
Benefits: Can be used to access DOM elements directly and store values across renders without causing additional renders.
useImperativeHandle
JavaScript
useImperativeHandle(ref, () => ({
focus: () => {
// focus logic
}
}));
Explanation: useImperativeHandle customizes the instance value that is exposed when using ref. Use it to pass methods to a parent component while using forwardRef.
Benefits: Allows parent components to invoke methods on child components.
useLayoutEffect
JavaScript
useLayoutEffect(() => {
// Read layout from the DOM and re-render synchronously
}, [dependencies]);
Explanation: useLayoutEffect works identically to useEffect, but it fires synchronously after all DOM mutations. Use this to read layout from the DOM and synchronously re-render.
Benefits: Ensures that updates are flushed synchronously before the browser has a chance to paint.
useDebugValue
JavaScript
useDebugValue(value);
Explanation: useDebugValue can be used to display a label for custom hooks in React DevTools.
Benefits: Easier debugging for custom hooks.
Conclusion React Hooks offer a powerful and expressive way to use React features without classes. They enable you to organize the logic inside a component into reusable isolated units, leading to cleaner and more maintainable codebases.
Benefits of Using React Hooks:
- Simplified Component Logic: Hooks allow you to split one component into smaller functions based on what pieces are related.
- Reusable Stateful Logic: Custom hooks let you reuse stateful logic without changing your component hierarchy.
- Improved Code Readability: By using hooks, your components and logic can be more declarative.
- Less Code: Hooks reduce the amount of code needed to achieve the same functionality compared to class components.
For more insights on freelancing, blogging, and creating your own website, check out my detailed guides:
Let’s get in touch
Get a risk-free, no obligation proposal in 1 business day or less.